Client-side functionalities
All set to go on communicating with apaleo’s UI? Before we jump into the funny part, let’s start with the basics.
Your app is running in an iframe. That means, that the parent of your Window Object
is apaleo.
Because of that, you can communicate with us in a message based way.
To achieve this, you have to use the following methods:
- postMessage method to send us a message
window.parent.postMessage(<your message>, "*")
We expect stringified JSON as a message. That means you have to convert your JSON / message to a string before you send it. For example,
window.parent.postMessage (JSON.stringify (<your message>), "*")
- addEventListener method to receive a message from us
window.addEventListener("message", <your callback>)
Response
The response which you can listen with window.addEventListener("message", <your callback>)
has the
following structure.
PROPERTY | DESCRIPTION |
---|---|
from | It is a hardcoded string [apaleo] . With this you will know that the response is from us. |
message | The message is a string which is used to give the user information about the problem or it can be a success message. |
extra | Extra can be anything. This is used to send you additional information. |
Here is a sample response:
{
"from": "[apaleo]",
"message": "A stringified JSON was expected but it was something else",
"extra": "<- here would be your message that you send ->"
}
Navigation
If you want to navigate in the apaleo UI without causing a reload, then you can send us this payload.
PROPERTY | DESCRIPTION |
---|---|
type | This is an identifier for us to know which type of message you send to us. For navigation messages it should be navigate . |
path | The path describes the navigation endpoint you want to go. |
context | Context can be account-level, property-level or both. |
id | The identifier is needed to differentiate between multiple navigation endpoints. As an example if you want to navigate to a reservation, then the id would be the reservation id. |
- account-level: You can only navigate in the account context. Allowed value:
$ACCOUNT
. - property-level: You can only navigate within properties. You can only navigate to properties where
you have access to. Allowed values:
$CURRENT_PROPERTY
or the property id. - both: It means that the path is available in account-level and property-level.
Endpoint details
Here you can see the available endpoints and in which context they can be used.
PATH | CONTEXT LEVEL | ID NEEDED | DESCRIPTION |
---|---|---|---|
apps | both | YES | Navigates to a UI Integration |
properties | account-level | - | Navigates to the properties overview in the account context |
reservation-details | property-level | YES | Navigates to the reservation details of a reservation |
Here is a sample payload to navigate to the reservation details of the reservation with the id “SOME-1” and property “AWE”:
{
"type": "navigate",
"path": "reservation-details",
"context": "AWE",
"id": "SOME-1",
}
Here is a sample payload to navigate to the reservation details of the reservation with the id “SOME-1” in the current property:
{
"type": "navigate",
"path": "reservation-details",
"context": "$CURRENT_PROPERTY",
"id": "SOME-1",
}
Notifications
Showing a notification is also easy. You can use the apaleo UI notification service to display any sort of message above your iframe, just as shown in the screen shot below.
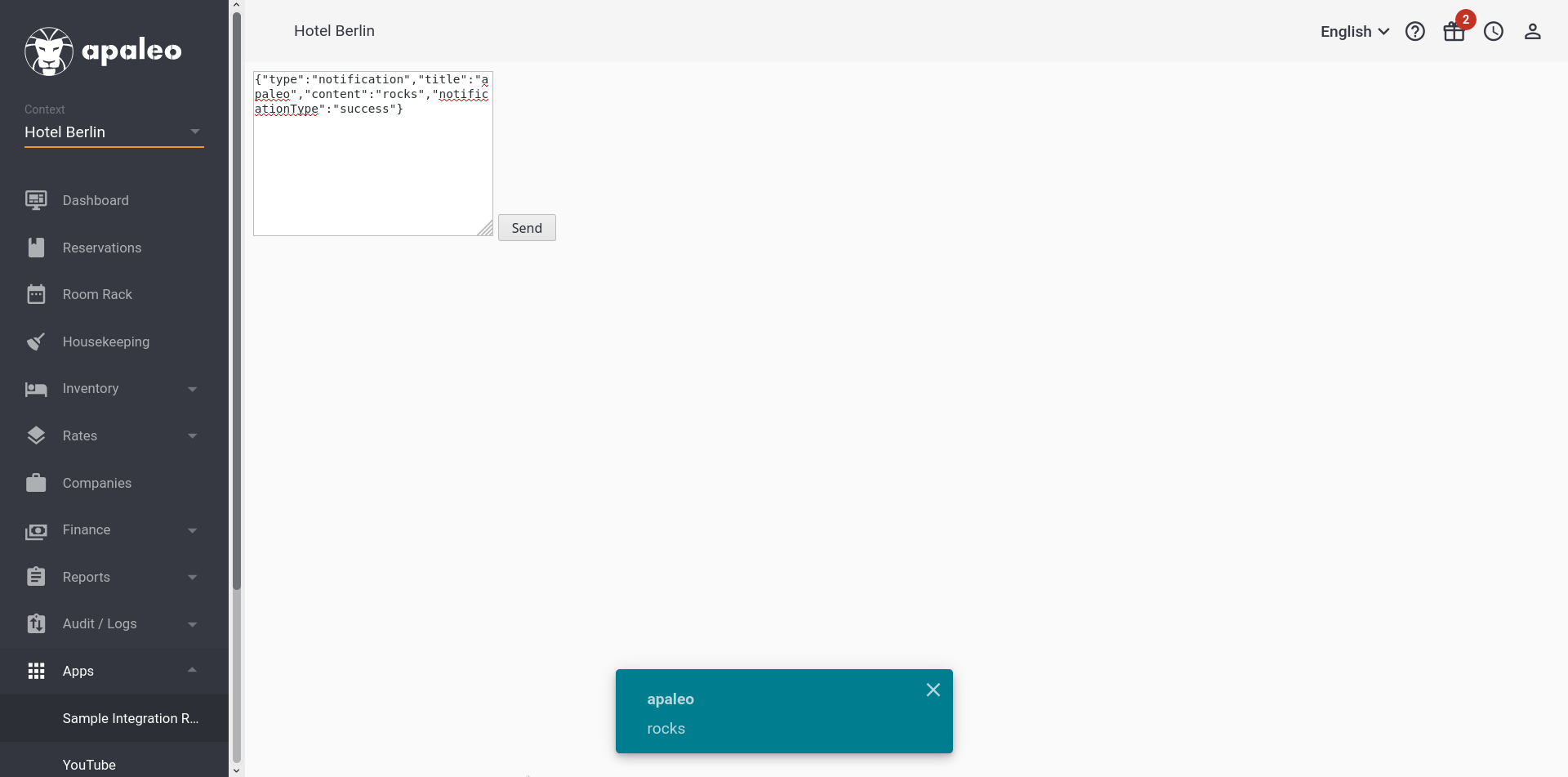
In order to do so, you can send us the following payload
Here is a sample payload to show a success notification:
{
"type": "notification",
"title": "apaleo",
"content": "rocks",
"notificationType": "success"
}
Properties
PROPERTY | OPTIONAL | DESCRIPTION |
---|---|---|
type | - | This is an identifier for us to know which type of message you send to us. For notification messages it should be notification . |
title | - | The title of the toast message. |
content | YES | The content of the toast message. |
notificationType | - | Type can be success, alert or error. |
Language and translations
In order for you to be able to manage the language of your integration, we provide the current
language of the apaleo UI to the iframe as a query parameter. So, if you want to translate your
integration so it is in the same language as the apaleo UI, you can use the lang
query parameter.
For example, when we load your integration into the iframe with your URL we provide the query parameters as follows
https://www.yourintegration.com?accountCode=[CurrentUserAccountCode]&lang=en&subjectId=[CurrentUserSubjectId]
As you can see the URL contains the query parameter lang=en
which you can use in your integration
in order to show in the desired language.